给定一个仅包含数字 2-9 的字符串,返回所有它能表示的字母组合。答案可以按任意顺序返回。给出数字到字母的映射如下(与电话按键相同)。注意 1 不对应任何字母。
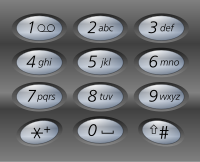
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| class Solution {
let letters = ["abc", "def", "ghi", "jkl", "mno", "pqrs", "tuv", "wxyz"]
func letterCombinations(_ digits: String) -> [String] { if digits.isEmpty { return [] } var res: [String] = [], temp: [Character] = [] backtrack(res: &res, temp: &temp, digits: Array(digits), index: 0) return res }
func backtrack(res: inout [String], temp: inout [Character], digits: [Character], index: Int) { guard index < digits.count else { return res.append(String(temp)) } let digit = digits[index].hexDigitValue! - 2
for val in letters[digit] { temp.append(val) backtrack(res: &res, temp: &temp, digits: digits, index: index + 1) temp.popLast() }
} }
|